Is there a way of reading the last element of an array with bash?
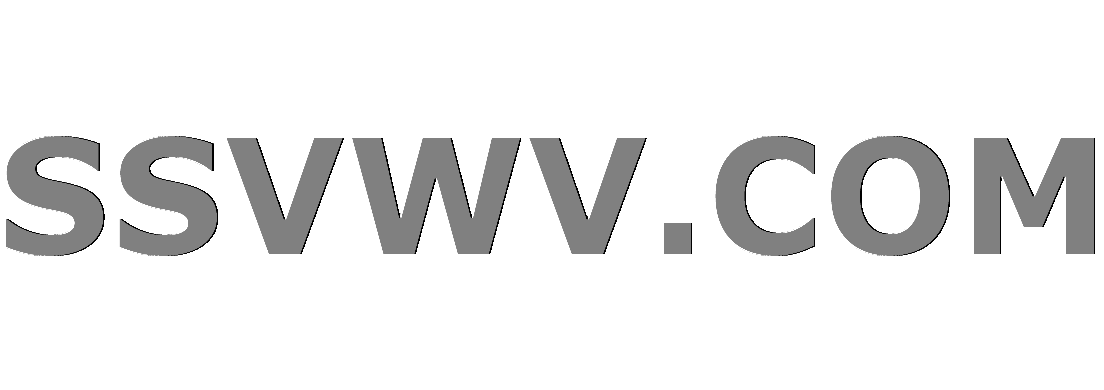
Multi tool use
If I have an array with 5 elements, for example:
[a][b][c][d][e]
Using echo ${myarray[4]}
I can see what it holds.
But what if I didn't know the number of elements in a given array? Is there a way of reading the last element of an unknown length array?
i.e. The first element reading from the right to the left for any array?
I would like to know how to do this in bash.
bash array
add a comment |
If I have an array with 5 elements, for example:
[a][b][c][d][e]
Using echo ${myarray[4]}
I can see what it holds.
But what if I didn't know the number of elements in a given array? Is there a way of reading the last element of an unknown length array?
i.e. The first element reading from the right to the left for any array?
I would like to know how to do this in bash.
bash array
add a comment |
If I have an array with 5 elements, for example:
[a][b][c][d][e]
Using echo ${myarray[4]}
I can see what it holds.
But what if I didn't know the number of elements in a given array? Is there a way of reading the last element of an unknown length array?
i.e. The first element reading from the right to the left for any array?
I would like to know how to do this in bash.
bash array
If I have an array with 5 elements, for example:
[a][b][c][d][e]
Using echo ${myarray[4]}
I can see what it holds.
But what if I didn't know the number of elements in a given array? Is there a way of reading the last element of an unknown length array?
i.e. The first element reading from the right to the left for any array?
I would like to know how to do this in bash.
bash array
bash array
edited Apr 27 '15 at 5:33


Mat
39.6k8121127
39.6k8121127
asked Apr 27 '15 at 4:37


3kstc3kstc
1,43072340
1,43072340
add a comment |
add a comment |
5 Answers
5
active
oldest
votes
You can just use a negative index ${myarray[-1]}
to get the last element. You can do the same thing for the second-last, and so on; in Bash:
If the subscript used to reference an element of an indexed array
evaluates to a number less than zero, it is interpreted as relative to
one greater than the maximum index of the array, so negative indices
count back from the end of the array, and an index of -1 refers to the
last element.
The same also works for assignment. When it says "expression" it really means an expression; you can write in any arithmetic expression there to compute the index, including one that computes using the length of the array ${#myarray[@]}
explicitly.
2
You can do that inksh
andzsh
as well.
– Janis
Apr 27 '15 at 4:43
5
Withzsh
though, by default arrays are 1-indexed, unlikebash
andksh
where they are 0-indexed.
– Stephen Kitt
Apr 27 '15 at 4:49
2
Yes, of course; the short answer to this question doesn't change, but since the long form was mentioned I thought it necessary to point out the difference in behaviour there.
– Stephen Kitt
Apr 27 '15 at 5:05
14
Negative index only work in bash 4.3 and above.
– cuonglm
Apr 27 '15 at 15:53
6
The version of Bash included with Mac OS X as of at least v10.11.5 is only 3.2, so this doesn't work on Macs.
– Doktor J
Nov 30 '16 at 23:05
|
show 2 more comments
Modern bash (v4.1 or better)
You can read the last element at index -1
:
$ a=(a b c d e f)
$ echo ${a[-1]}
f
Support for accessing numerically-indexed arrays from the end using negative indexes started with bash version 4.1-alpha.
Older bash (v4.0 or earlier)
You must get the array length from ${#a[@]}
and then subtract one to get the last element:
$ echo ${a[${#a[@]}-1]}
f
Since bash treats array subscripts as an arithmetic expression, there is no need for additional notation, such as $((...))
, to force arithmetic evaluation.
the last one doesn't work for me; I'm using Bash v4.1.2(1): instead of printing the last item, it just prints out the whole array.
– Alexej Magura
Oct 4 '16 at 15:34
@cuonglm's answer works, however.
– Alexej Magura
Oct 4 '16 at 15:53
The answer would be even better if you could qualifymodern
with a version.
– Samveen
May 16 '17 at 3:08
1
Exactly what was needed to make the anwser perfect.
– Samveen
May 17 '17 at 9:40
1
Thank you for this. I was using echo ${a[$((${#a[@]}-1]))} because I didn't know about "bash treats array subscripts as an arithmetic expression".
– Bruno Bronosky
Dec 21 '18 at 6:29
|
show 1 more comment
bash
array assignment, reference, unsetting with negative index were only added in bash 4.3. With older version of bash
, you can use expression in index array[${#array[@]-1}]
Another way, also work with older version of bash
(bash 3.0 or better):
$ a=([a] [b] [c] [d] [e])
$ printf %s\n "${a[@]:(-1)}"
[e]
or:
$ printf %s\n "${a[@]: -1}"
[e]
Using negative offset, you need to separate :
with -
to avoid being confused with the :-
expansion.
1
Make that"${a[@]: -1}"
and it will work (besidesbash
andzsh
) also inksh
.
– Janis
Apr 27 '15 at 5:09
The Kornshell docs (www2.research.att.com/sw/download/man/man1/ksh.html) specify it completely. (Haven't inspected the docs ofzsh
orbash
; but I tested it in all three shells.)
– Janis
Apr 27 '15 at 5:20
@Janis: re-read bash documentation, it also mentioned about this one, too. Thanks again.
– cuonglm
Apr 27 '15 at 5:21
@cuonglm there is a small bug, however, it seems to only be capable of grabbing the last character from the last item in an array:a.sh e a f gh
->h
(even whengh
is quoted'gh'
only theh
gets grabbed)
– Alexej Magura
Oct 4 '16 at 19:08
add a comment |
Also you can do this:
$ a=(a b c d e f)
$ echo ${a[$(expr ${#a[@]} - 1)]}
Result:
$ f
What you're doing is getting all the count of elements in the array and subtract -1 due you're getting all the elements, not starting from the array index that is 0.
add a comment |
The oldest alternative in bash (Since bash 3.0+) is:
$ a=(a b c d e)
$ echo "${a[@]:(-1)}"
e
Or:
$ echo "${a[@]:(~0)}"
e
In bash-4.2+ :
$ echo "${a[-1]}" # or "${a[(~0)]}"
e
In bash 5.0+ :
$ echo "${a[~0]}"
e
For older bash (all bash versions):
$ echo "${a[${#a[@]}-1]}"
e
add a comment |
Your Answer
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "106"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2funix.stackexchange.com%2fquestions%2f198787%2fis-there-a-way-of-reading-the-last-element-of-an-array-with-bash%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can just use a negative index ${myarray[-1]}
to get the last element. You can do the same thing for the second-last, and so on; in Bash:
If the subscript used to reference an element of an indexed array
evaluates to a number less than zero, it is interpreted as relative to
one greater than the maximum index of the array, so negative indices
count back from the end of the array, and an index of -1 refers to the
last element.
The same also works for assignment. When it says "expression" it really means an expression; you can write in any arithmetic expression there to compute the index, including one that computes using the length of the array ${#myarray[@]}
explicitly.
2
You can do that inksh
andzsh
as well.
– Janis
Apr 27 '15 at 4:43
5
Withzsh
though, by default arrays are 1-indexed, unlikebash
andksh
where they are 0-indexed.
– Stephen Kitt
Apr 27 '15 at 4:49
2
Yes, of course; the short answer to this question doesn't change, but since the long form was mentioned I thought it necessary to point out the difference in behaviour there.
– Stephen Kitt
Apr 27 '15 at 5:05
14
Negative index only work in bash 4.3 and above.
– cuonglm
Apr 27 '15 at 15:53
6
The version of Bash included with Mac OS X as of at least v10.11.5 is only 3.2, so this doesn't work on Macs.
– Doktor J
Nov 30 '16 at 23:05
|
show 2 more comments
You can just use a negative index ${myarray[-1]}
to get the last element. You can do the same thing for the second-last, and so on; in Bash:
If the subscript used to reference an element of an indexed array
evaluates to a number less than zero, it is interpreted as relative to
one greater than the maximum index of the array, so negative indices
count back from the end of the array, and an index of -1 refers to the
last element.
The same also works for assignment. When it says "expression" it really means an expression; you can write in any arithmetic expression there to compute the index, including one that computes using the length of the array ${#myarray[@]}
explicitly.
2
You can do that inksh
andzsh
as well.
– Janis
Apr 27 '15 at 4:43
5
Withzsh
though, by default arrays are 1-indexed, unlikebash
andksh
where they are 0-indexed.
– Stephen Kitt
Apr 27 '15 at 4:49
2
Yes, of course; the short answer to this question doesn't change, but since the long form was mentioned I thought it necessary to point out the difference in behaviour there.
– Stephen Kitt
Apr 27 '15 at 5:05
14
Negative index only work in bash 4.3 and above.
– cuonglm
Apr 27 '15 at 15:53
6
The version of Bash included with Mac OS X as of at least v10.11.5 is only 3.2, so this doesn't work on Macs.
– Doktor J
Nov 30 '16 at 23:05
|
show 2 more comments
You can just use a negative index ${myarray[-1]}
to get the last element. You can do the same thing for the second-last, and so on; in Bash:
If the subscript used to reference an element of an indexed array
evaluates to a number less than zero, it is interpreted as relative to
one greater than the maximum index of the array, so negative indices
count back from the end of the array, and an index of -1 refers to the
last element.
The same also works for assignment. When it says "expression" it really means an expression; you can write in any arithmetic expression there to compute the index, including one that computes using the length of the array ${#myarray[@]}
explicitly.
You can just use a negative index ${myarray[-1]}
to get the last element. You can do the same thing for the second-last, and so on; in Bash:
If the subscript used to reference an element of an indexed array
evaluates to a number less than zero, it is interpreted as relative to
one greater than the maximum index of the array, so negative indices
count back from the end of the array, and an index of -1 refers to the
last element.
The same also works for assignment. When it says "expression" it really means an expression; you can write in any arithmetic expression there to compute the index, including one that computes using the length of the array ${#myarray[@]}
explicitly.
edited Apr 27 '15 at 4:44
answered Apr 27 '15 at 4:41


Michael HomerMichael Homer
49.2k8133172
49.2k8133172
2
You can do that inksh
andzsh
as well.
– Janis
Apr 27 '15 at 4:43
5
Withzsh
though, by default arrays are 1-indexed, unlikebash
andksh
where they are 0-indexed.
– Stephen Kitt
Apr 27 '15 at 4:49
2
Yes, of course; the short answer to this question doesn't change, but since the long form was mentioned I thought it necessary to point out the difference in behaviour there.
– Stephen Kitt
Apr 27 '15 at 5:05
14
Negative index only work in bash 4.3 and above.
– cuonglm
Apr 27 '15 at 15:53
6
The version of Bash included with Mac OS X as of at least v10.11.5 is only 3.2, so this doesn't work on Macs.
– Doktor J
Nov 30 '16 at 23:05
|
show 2 more comments
2
You can do that inksh
andzsh
as well.
– Janis
Apr 27 '15 at 4:43
5
Withzsh
though, by default arrays are 1-indexed, unlikebash
andksh
where they are 0-indexed.
– Stephen Kitt
Apr 27 '15 at 4:49
2
Yes, of course; the short answer to this question doesn't change, but since the long form was mentioned I thought it necessary to point out the difference in behaviour there.
– Stephen Kitt
Apr 27 '15 at 5:05
14
Negative index only work in bash 4.3 and above.
– cuonglm
Apr 27 '15 at 15:53
6
The version of Bash included with Mac OS X as of at least v10.11.5 is only 3.2, so this doesn't work on Macs.
– Doktor J
Nov 30 '16 at 23:05
2
2
You can do that in
ksh
and zsh
as well.– Janis
Apr 27 '15 at 4:43
You can do that in
ksh
and zsh
as well.– Janis
Apr 27 '15 at 4:43
5
5
With
zsh
though, by default arrays are 1-indexed, unlike bash
and ksh
where they are 0-indexed.– Stephen Kitt
Apr 27 '15 at 4:49
With
zsh
though, by default arrays are 1-indexed, unlike bash
and ksh
where they are 0-indexed.– Stephen Kitt
Apr 27 '15 at 4:49
2
2
Yes, of course; the short answer to this question doesn't change, but since the long form was mentioned I thought it necessary to point out the difference in behaviour there.
– Stephen Kitt
Apr 27 '15 at 5:05
Yes, of course; the short answer to this question doesn't change, but since the long form was mentioned I thought it necessary to point out the difference in behaviour there.
– Stephen Kitt
Apr 27 '15 at 5:05
14
14
Negative index only work in bash 4.3 and above.
– cuonglm
Apr 27 '15 at 15:53
Negative index only work in bash 4.3 and above.
– cuonglm
Apr 27 '15 at 15:53
6
6
The version of Bash included with Mac OS X as of at least v10.11.5 is only 3.2, so this doesn't work on Macs.
– Doktor J
Nov 30 '16 at 23:05
The version of Bash included with Mac OS X as of at least v10.11.5 is only 3.2, so this doesn't work on Macs.
– Doktor J
Nov 30 '16 at 23:05
|
show 2 more comments
Modern bash (v4.1 or better)
You can read the last element at index -1
:
$ a=(a b c d e f)
$ echo ${a[-1]}
f
Support for accessing numerically-indexed arrays from the end using negative indexes started with bash version 4.1-alpha.
Older bash (v4.0 or earlier)
You must get the array length from ${#a[@]}
and then subtract one to get the last element:
$ echo ${a[${#a[@]}-1]}
f
Since bash treats array subscripts as an arithmetic expression, there is no need for additional notation, such as $((...))
, to force arithmetic evaluation.
the last one doesn't work for me; I'm using Bash v4.1.2(1): instead of printing the last item, it just prints out the whole array.
– Alexej Magura
Oct 4 '16 at 15:34
@cuonglm's answer works, however.
– Alexej Magura
Oct 4 '16 at 15:53
The answer would be even better if you could qualifymodern
with a version.
– Samveen
May 16 '17 at 3:08
1
Exactly what was needed to make the anwser perfect.
– Samveen
May 17 '17 at 9:40
1
Thank you for this. I was using echo ${a[$((${#a[@]}-1]))} because I didn't know about "bash treats array subscripts as an arithmetic expression".
– Bruno Bronosky
Dec 21 '18 at 6:29
|
show 1 more comment
Modern bash (v4.1 or better)
You can read the last element at index -1
:
$ a=(a b c d e f)
$ echo ${a[-1]}
f
Support for accessing numerically-indexed arrays from the end using negative indexes started with bash version 4.1-alpha.
Older bash (v4.0 or earlier)
You must get the array length from ${#a[@]}
and then subtract one to get the last element:
$ echo ${a[${#a[@]}-1]}
f
Since bash treats array subscripts as an arithmetic expression, there is no need for additional notation, such as $((...))
, to force arithmetic evaluation.
the last one doesn't work for me; I'm using Bash v4.1.2(1): instead of printing the last item, it just prints out the whole array.
– Alexej Magura
Oct 4 '16 at 15:34
@cuonglm's answer works, however.
– Alexej Magura
Oct 4 '16 at 15:53
The answer would be even better if you could qualifymodern
with a version.
– Samveen
May 16 '17 at 3:08
1
Exactly what was needed to make the anwser perfect.
– Samveen
May 17 '17 at 9:40
1
Thank you for this. I was using echo ${a[$((${#a[@]}-1]))} because I didn't know about "bash treats array subscripts as an arithmetic expression".
– Bruno Bronosky
Dec 21 '18 at 6:29
|
show 1 more comment
Modern bash (v4.1 or better)
You can read the last element at index -1
:
$ a=(a b c d e f)
$ echo ${a[-1]}
f
Support for accessing numerically-indexed arrays from the end using negative indexes started with bash version 4.1-alpha.
Older bash (v4.0 or earlier)
You must get the array length from ${#a[@]}
and then subtract one to get the last element:
$ echo ${a[${#a[@]}-1]}
f
Since bash treats array subscripts as an arithmetic expression, there is no need for additional notation, such as $((...))
, to force arithmetic evaluation.
Modern bash (v4.1 or better)
You can read the last element at index -1
:
$ a=(a b c d e f)
$ echo ${a[-1]}
f
Support for accessing numerically-indexed arrays from the end using negative indexes started with bash version 4.1-alpha.
Older bash (v4.0 or earlier)
You must get the array length from ${#a[@]}
and then subtract one to get the last element:
$ echo ${a[${#a[@]}-1]}
f
Since bash treats array subscripts as an arithmetic expression, there is no need for additional notation, such as $((...))
, to force arithmetic evaluation.
edited Apr 11 '18 at 21:33
answered Apr 27 '15 at 4:40


John1024John1024
47.3k5110125
47.3k5110125
the last one doesn't work for me; I'm using Bash v4.1.2(1): instead of printing the last item, it just prints out the whole array.
– Alexej Magura
Oct 4 '16 at 15:34
@cuonglm's answer works, however.
– Alexej Magura
Oct 4 '16 at 15:53
The answer would be even better if you could qualifymodern
with a version.
– Samveen
May 16 '17 at 3:08
1
Exactly what was needed to make the anwser perfect.
– Samveen
May 17 '17 at 9:40
1
Thank you for this. I was using echo ${a[$((${#a[@]}-1]))} because I didn't know about "bash treats array subscripts as an arithmetic expression".
– Bruno Bronosky
Dec 21 '18 at 6:29
|
show 1 more comment
the last one doesn't work for me; I'm using Bash v4.1.2(1): instead of printing the last item, it just prints out the whole array.
– Alexej Magura
Oct 4 '16 at 15:34
@cuonglm's answer works, however.
– Alexej Magura
Oct 4 '16 at 15:53
The answer would be even better if you could qualifymodern
with a version.
– Samveen
May 16 '17 at 3:08
1
Exactly what was needed to make the anwser perfect.
– Samveen
May 17 '17 at 9:40
1
Thank you for this. I was using echo ${a[$((${#a[@]}-1]))} because I didn't know about "bash treats array subscripts as an arithmetic expression".
– Bruno Bronosky
Dec 21 '18 at 6:29
the last one doesn't work for me; I'm using Bash v4.1.2(1): instead of printing the last item, it just prints out the whole array.
– Alexej Magura
Oct 4 '16 at 15:34
the last one doesn't work for me; I'm using Bash v4.1.2(1): instead of printing the last item, it just prints out the whole array.
– Alexej Magura
Oct 4 '16 at 15:34
@cuonglm's answer works, however.
– Alexej Magura
Oct 4 '16 at 15:53
@cuonglm's answer works, however.
– Alexej Magura
Oct 4 '16 at 15:53
The answer would be even better if you could qualify
modern
with a version.– Samveen
May 16 '17 at 3:08
The answer would be even better if you could qualify
modern
with a version.– Samveen
May 16 '17 at 3:08
1
1
Exactly what was needed to make the anwser perfect.
– Samveen
May 17 '17 at 9:40
Exactly what was needed to make the anwser perfect.
– Samveen
May 17 '17 at 9:40
1
1
Thank you for this. I was using echo ${a[$((${#a[@]}-1]))} because I didn't know about "bash treats array subscripts as an arithmetic expression".
– Bruno Bronosky
Dec 21 '18 at 6:29
Thank you for this. I was using echo ${a[$((${#a[@]}-1]))} because I didn't know about "bash treats array subscripts as an arithmetic expression".
– Bruno Bronosky
Dec 21 '18 at 6:29
|
show 1 more comment
bash
array assignment, reference, unsetting with negative index were only added in bash 4.3. With older version of bash
, you can use expression in index array[${#array[@]-1}]
Another way, also work with older version of bash
(bash 3.0 or better):
$ a=([a] [b] [c] [d] [e])
$ printf %s\n "${a[@]:(-1)}"
[e]
or:
$ printf %s\n "${a[@]: -1}"
[e]
Using negative offset, you need to separate :
with -
to avoid being confused with the :-
expansion.
1
Make that"${a[@]: -1}"
and it will work (besidesbash
andzsh
) also inksh
.
– Janis
Apr 27 '15 at 5:09
The Kornshell docs (www2.research.att.com/sw/download/man/man1/ksh.html) specify it completely. (Haven't inspected the docs ofzsh
orbash
; but I tested it in all three shells.)
– Janis
Apr 27 '15 at 5:20
@Janis: re-read bash documentation, it also mentioned about this one, too. Thanks again.
– cuonglm
Apr 27 '15 at 5:21
@cuonglm there is a small bug, however, it seems to only be capable of grabbing the last character from the last item in an array:a.sh e a f gh
->h
(even whengh
is quoted'gh'
only theh
gets grabbed)
– Alexej Magura
Oct 4 '16 at 19:08
add a comment |
bash
array assignment, reference, unsetting with negative index were only added in bash 4.3. With older version of bash
, you can use expression in index array[${#array[@]-1}]
Another way, also work with older version of bash
(bash 3.0 or better):
$ a=([a] [b] [c] [d] [e])
$ printf %s\n "${a[@]:(-1)}"
[e]
or:
$ printf %s\n "${a[@]: -1}"
[e]
Using negative offset, you need to separate :
with -
to avoid being confused with the :-
expansion.
1
Make that"${a[@]: -1}"
and it will work (besidesbash
andzsh
) also inksh
.
– Janis
Apr 27 '15 at 5:09
The Kornshell docs (www2.research.att.com/sw/download/man/man1/ksh.html) specify it completely. (Haven't inspected the docs ofzsh
orbash
; but I tested it in all three shells.)
– Janis
Apr 27 '15 at 5:20
@Janis: re-read bash documentation, it also mentioned about this one, too. Thanks again.
– cuonglm
Apr 27 '15 at 5:21
@cuonglm there is a small bug, however, it seems to only be capable of grabbing the last character from the last item in an array:a.sh e a f gh
->h
(even whengh
is quoted'gh'
only theh
gets grabbed)
– Alexej Magura
Oct 4 '16 at 19:08
add a comment |
bash
array assignment, reference, unsetting with negative index were only added in bash 4.3. With older version of bash
, you can use expression in index array[${#array[@]-1}]
Another way, also work with older version of bash
(bash 3.0 or better):
$ a=([a] [b] [c] [d] [e])
$ printf %s\n "${a[@]:(-1)}"
[e]
or:
$ printf %s\n "${a[@]: -1}"
[e]
Using negative offset, you need to separate :
with -
to avoid being confused with the :-
expansion.
bash
array assignment, reference, unsetting with negative index were only added in bash 4.3. With older version of bash
, you can use expression in index array[${#array[@]-1}]
Another way, also work with older version of bash
(bash 3.0 or better):
$ a=([a] [b] [c] [d] [e])
$ printf %s\n "${a[@]:(-1)}"
[e]
or:
$ printf %s\n "${a[@]: -1}"
[e]
Using negative offset, you need to separate :
with -
to avoid being confused with the :-
expansion.
edited Dec 21 '18 at 7:51
Bruno Bronosky
1,93711112
1,93711112
answered Apr 27 '15 at 4:50
cuonglmcuonglm
104k25206303
104k25206303
1
Make that"${a[@]: -1}"
and it will work (besidesbash
andzsh
) also inksh
.
– Janis
Apr 27 '15 at 5:09
The Kornshell docs (www2.research.att.com/sw/download/man/man1/ksh.html) specify it completely. (Haven't inspected the docs ofzsh
orbash
; but I tested it in all three shells.)
– Janis
Apr 27 '15 at 5:20
@Janis: re-read bash documentation, it also mentioned about this one, too. Thanks again.
– cuonglm
Apr 27 '15 at 5:21
@cuonglm there is a small bug, however, it seems to only be capable of grabbing the last character from the last item in an array:a.sh e a f gh
->h
(even whengh
is quoted'gh'
only theh
gets grabbed)
– Alexej Magura
Oct 4 '16 at 19:08
add a comment |
1
Make that"${a[@]: -1}"
and it will work (besidesbash
andzsh
) also inksh
.
– Janis
Apr 27 '15 at 5:09
The Kornshell docs (www2.research.att.com/sw/download/man/man1/ksh.html) specify it completely. (Haven't inspected the docs ofzsh
orbash
; but I tested it in all three shells.)
– Janis
Apr 27 '15 at 5:20
@Janis: re-read bash documentation, it also mentioned about this one, too. Thanks again.
– cuonglm
Apr 27 '15 at 5:21
@cuonglm there is a small bug, however, it seems to only be capable of grabbing the last character from the last item in an array:a.sh e a f gh
->h
(even whengh
is quoted'gh'
only theh
gets grabbed)
– Alexej Magura
Oct 4 '16 at 19:08
1
1
Make that
"${a[@]: -1}"
and it will work (besides bash
and zsh
) also in ksh
.– Janis
Apr 27 '15 at 5:09
Make that
"${a[@]: -1}"
and it will work (besides bash
and zsh
) also in ksh
.– Janis
Apr 27 '15 at 5:09
The Kornshell docs (www2.research.att.com/sw/download/man/man1/ksh.html) specify it completely. (Haven't inspected the docs of
zsh
or bash
; but I tested it in all three shells.)– Janis
Apr 27 '15 at 5:20
The Kornshell docs (www2.research.att.com/sw/download/man/man1/ksh.html) specify it completely. (Haven't inspected the docs of
zsh
or bash
; but I tested it in all three shells.)– Janis
Apr 27 '15 at 5:20
@Janis: re-read bash documentation, it also mentioned about this one, too. Thanks again.
– cuonglm
Apr 27 '15 at 5:21
@Janis: re-read bash documentation, it also mentioned about this one, too. Thanks again.
– cuonglm
Apr 27 '15 at 5:21
@cuonglm there is a small bug, however, it seems to only be capable of grabbing the last character from the last item in an array:
a.sh e a f gh
-> h
(even when gh
is quoted 'gh'
only the h
gets grabbed)– Alexej Magura
Oct 4 '16 at 19:08
@cuonglm there is a small bug, however, it seems to only be capable of grabbing the last character from the last item in an array:
a.sh e a f gh
-> h
(even when gh
is quoted 'gh'
only the h
gets grabbed)– Alexej Magura
Oct 4 '16 at 19:08
add a comment |
Also you can do this:
$ a=(a b c d e f)
$ echo ${a[$(expr ${#a[@]} - 1)]}
Result:
$ f
What you're doing is getting all the count of elements in the array and subtract -1 due you're getting all the elements, not starting from the array index that is 0.
add a comment |
Also you can do this:
$ a=(a b c d e f)
$ echo ${a[$(expr ${#a[@]} - 1)]}
Result:
$ f
What you're doing is getting all the count of elements in the array and subtract -1 due you're getting all the elements, not starting from the array index that is 0.
add a comment |
Also you can do this:
$ a=(a b c d e f)
$ echo ${a[$(expr ${#a[@]} - 1)]}
Result:
$ f
What you're doing is getting all the count of elements in the array and subtract -1 due you're getting all the elements, not starting from the array index that is 0.
Also you can do this:
$ a=(a b c d e f)
$ echo ${a[$(expr ${#a[@]} - 1)]}
Result:
$ f
What you're doing is getting all the count of elements in the array and subtract -1 due you're getting all the elements, not starting from the array index that is 0.
answered Mar 13 '17 at 20:43
Javier SalasJavier Salas
1266
1266
add a comment |
add a comment |
The oldest alternative in bash (Since bash 3.0+) is:
$ a=(a b c d e)
$ echo "${a[@]:(-1)}"
e
Or:
$ echo "${a[@]:(~0)}"
e
In bash-4.2+ :
$ echo "${a[-1]}" # or "${a[(~0)]}"
e
In bash 5.0+ :
$ echo "${a[~0]}"
e
For older bash (all bash versions):
$ echo "${a[${#a[@]}-1]}"
e
add a comment |
The oldest alternative in bash (Since bash 3.0+) is:
$ a=(a b c d e)
$ echo "${a[@]:(-1)}"
e
Or:
$ echo "${a[@]:(~0)}"
e
In bash-4.2+ :
$ echo "${a[-1]}" # or "${a[(~0)]}"
e
In bash 5.0+ :
$ echo "${a[~0]}"
e
For older bash (all bash versions):
$ echo "${a[${#a[@]}-1]}"
e
add a comment |
The oldest alternative in bash (Since bash 3.0+) is:
$ a=(a b c d e)
$ echo "${a[@]:(-1)}"
e
Or:
$ echo "${a[@]:(~0)}"
e
In bash-4.2+ :
$ echo "${a[-1]}" # or "${a[(~0)]}"
e
In bash 5.0+ :
$ echo "${a[~0]}"
e
For older bash (all bash versions):
$ echo "${a[${#a[@]}-1]}"
e
The oldest alternative in bash (Since bash 3.0+) is:
$ a=(a b c d e)
$ echo "${a[@]:(-1)}"
e
Or:
$ echo "${a[@]:(~0)}"
e
In bash-4.2+ :
$ echo "${a[-1]}" # or "${a[(~0)]}"
e
In bash 5.0+ :
$ echo "${a[~0]}"
e
For older bash (all bash versions):
$ echo "${a[${#a[@]}-1]}"
e
answered 45 mins ago


IsaacIsaac
12k11852
12k11852
add a comment |
add a comment |
Thanks for contributing an answer to Unix & Linux Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2funix.stackexchange.com%2fquestions%2f198787%2fis-there-a-way-of-reading-the-last-element-of-an-array-with-bash%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
LlaL0tdgYCl,zfGpI0 c,WlX 8VrinOFblyXwG6h ALSUk,ljCPW GSshRxkx1un,7OZ aDf txf94r8NvH ChDUioOUqtyvxVm7zi