Writing an awk script that reads variable from command line
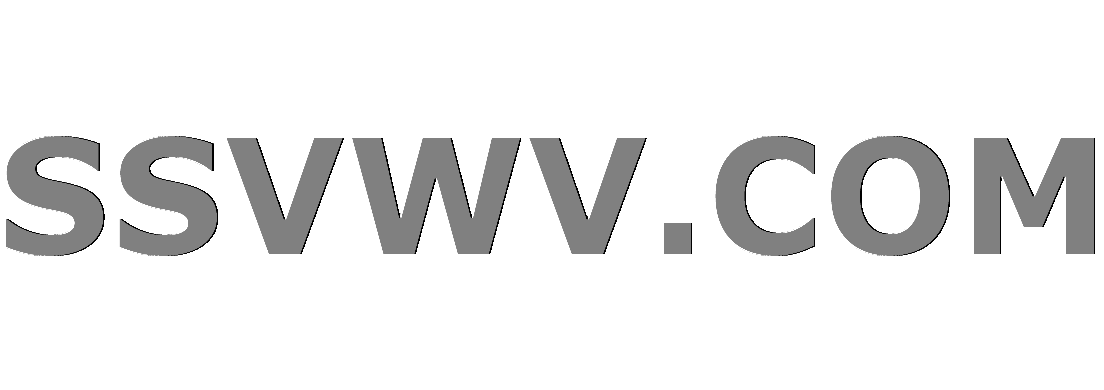
Multi tool use
I am trying to write an awk
script that can read a users input from command line and store it as a variable.
I know that if I compile from command line and do
./myawk.awk -v ID=4 text.sql
ID will be a variable set to 4. However, the input test cases I am given for my script are just
./myawk.awk ID=4 text.sql
The final goal of the script is to take an ID, find any line that begins with
-- ID
and print the following line. What I have so far is
#!/bin/awk -f
{
if ($1 ~/--/ && $2 ~/^ID/){
x = NR+1
}
while (NR <= x){
print $0
}
}
Can someone explain to me how I am able to read the variable ID without altering the line and adding -v
before it, as well maybe give me advice on my current awk
script.
awk
New contributor
Connor Hale is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
I am trying to write an awk
script that can read a users input from command line and store it as a variable.
I know that if I compile from command line and do
./myawk.awk -v ID=4 text.sql
ID will be a variable set to 4. However, the input test cases I am given for my script are just
./myawk.awk ID=4 text.sql
The final goal of the script is to take an ID, find any line that begins with
-- ID
and print the following line. What I have so far is
#!/bin/awk -f
{
if ($1 ~/--/ && $2 ~/^ID/){
x = NR+1
}
while (NR <= x){
print $0
}
}
Can someone explain to me how I am able to read the variable ID without altering the line and adding -v
before it, as well maybe give me advice on my current awk
script.
awk
New contributor
Connor Hale is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
I am trying to write an awk
script that can read a users input from command line and store it as a variable.
I know that if I compile from command line and do
./myawk.awk -v ID=4 text.sql
ID will be a variable set to 4. However, the input test cases I am given for my script are just
./myawk.awk ID=4 text.sql
The final goal of the script is to take an ID, find any line that begins with
-- ID
and print the following line. What I have so far is
#!/bin/awk -f
{
if ($1 ~/--/ && $2 ~/^ID/){
x = NR+1
}
while (NR <= x){
print $0
}
}
Can someone explain to me how I am able to read the variable ID without altering the line and adding -v
before it, as well maybe give me advice on my current awk
script.
awk
New contributor
Connor Hale is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
I am trying to write an awk
script that can read a users input from command line and store it as a variable.
I know that if I compile from command line and do
./myawk.awk -v ID=4 text.sql
ID will be a variable set to 4. However, the input test cases I am given for my script are just
./myawk.awk ID=4 text.sql
The final goal of the script is to take an ID, find any line that begins with
-- ID
and print the following line. What I have so far is
#!/bin/awk -f
{
if ($1 ~/--/ && $2 ~/^ID/){
x = NR+1
}
while (NR <= x){
print $0
}
}
Can someone explain to me how I am able to read the variable ID without altering the line and adding -v
before it, as well maybe give me advice on my current awk
script.
awk
awk
New contributor
Connor Hale is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Connor Hale is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited 1 hour ago


Inian
4,5851026
4,5851026
New contributor
Connor Hale is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 1 hour ago


Connor HaleConnor Hale
1
1
New contributor
Connor Hale is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Connor Hale is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Connor Hale is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
You just need to use the ENVIRON
function to use environment variables set from the shell. Import it once in the BEGIN
clause and use the variable as you need in the rest of the script.
#!/bin/awk -f
BEGIN {
argID=ENVIRON["ID"]
}
add a comment |
If a variable assignment is given as a file operand on the command line of an awk
script, that assignment will be carried out when awk
gets to that point in using the file operands.
Example:
awk -f script.awk a=1 file1 file2 a=2 file3 a=3 file4
Here, the awk
variable a
will be set to 1
before reading file1
. The variable will retain that value (unless it's changed by the awk
program) until the file file2
has been read. It is then set to 2
etc.
This means that your command line properly sets the variable ID
to 4
.
The difference between -v ID=4
and this is that ID
will not have been set in any BEGIN
block. Using -v ID=4
sets ID
to 4
and it will be available in BEGIN
blocks.
Another issue with your code is that you use ID
as a literal string in
if ($1 ~/--/ && $2 ~/^ID/){
And also that you seem to expect your while
loop to loop over the lines of the file. That line-by-line reading loop is already built into the way awk
operates and it very seldom needs to be explicit.
Instead,
$1 == "--" && $2 == ID { getline; print }
This will use string comparisons (not regular expression matches) to check whether the first whitespace-delimited field on the current line is the string --
and whether the second such field is the value of the variable ID
. If so, the next line of input is read and printed.
To use regular expressions instead,
$0 ~ "^-- " ID { getline; print }
This would match every line that starts with a double dash and a single space, followed by the value of ID
.
add a comment |
Your Answer
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "106"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Connor Hale is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2funix.stackexchange.com%2fquestions%2f503047%2fwriting-an-awk-script-that-reads-variable-from-command-line%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You just need to use the ENVIRON
function to use environment variables set from the shell. Import it once in the BEGIN
clause and use the variable as you need in the rest of the script.
#!/bin/awk -f
BEGIN {
argID=ENVIRON["ID"]
}
add a comment |
You just need to use the ENVIRON
function to use environment variables set from the shell. Import it once in the BEGIN
clause and use the variable as you need in the rest of the script.
#!/bin/awk -f
BEGIN {
argID=ENVIRON["ID"]
}
add a comment |
You just need to use the ENVIRON
function to use environment variables set from the shell. Import it once in the BEGIN
clause and use the variable as you need in the rest of the script.
#!/bin/awk -f
BEGIN {
argID=ENVIRON["ID"]
}
You just need to use the ENVIRON
function to use environment variables set from the shell. Import it once in the BEGIN
clause and use the variable as you need in the rest of the script.
#!/bin/awk -f
BEGIN {
argID=ENVIRON["ID"]
}
answered 1 hour ago


InianInian
4,5851026
4,5851026
add a comment |
add a comment |
If a variable assignment is given as a file operand on the command line of an awk
script, that assignment will be carried out when awk
gets to that point in using the file operands.
Example:
awk -f script.awk a=1 file1 file2 a=2 file3 a=3 file4
Here, the awk
variable a
will be set to 1
before reading file1
. The variable will retain that value (unless it's changed by the awk
program) until the file file2
has been read. It is then set to 2
etc.
This means that your command line properly sets the variable ID
to 4
.
The difference between -v ID=4
and this is that ID
will not have been set in any BEGIN
block. Using -v ID=4
sets ID
to 4
and it will be available in BEGIN
blocks.
Another issue with your code is that you use ID
as a literal string in
if ($1 ~/--/ && $2 ~/^ID/){
And also that you seem to expect your while
loop to loop over the lines of the file. That line-by-line reading loop is already built into the way awk
operates and it very seldom needs to be explicit.
Instead,
$1 == "--" && $2 == ID { getline; print }
This will use string comparisons (not regular expression matches) to check whether the first whitespace-delimited field on the current line is the string --
and whether the second such field is the value of the variable ID
. If so, the next line of input is read and printed.
To use regular expressions instead,
$0 ~ "^-- " ID { getline; print }
This would match every line that starts with a double dash and a single space, followed by the value of ID
.
add a comment |
If a variable assignment is given as a file operand on the command line of an awk
script, that assignment will be carried out when awk
gets to that point in using the file operands.
Example:
awk -f script.awk a=1 file1 file2 a=2 file3 a=3 file4
Here, the awk
variable a
will be set to 1
before reading file1
. The variable will retain that value (unless it's changed by the awk
program) until the file file2
has been read. It is then set to 2
etc.
This means that your command line properly sets the variable ID
to 4
.
The difference between -v ID=4
and this is that ID
will not have been set in any BEGIN
block. Using -v ID=4
sets ID
to 4
and it will be available in BEGIN
blocks.
Another issue with your code is that you use ID
as a literal string in
if ($1 ~/--/ && $2 ~/^ID/){
And also that you seem to expect your while
loop to loop over the lines of the file. That line-by-line reading loop is already built into the way awk
operates and it very seldom needs to be explicit.
Instead,
$1 == "--" && $2 == ID { getline; print }
This will use string comparisons (not regular expression matches) to check whether the first whitespace-delimited field on the current line is the string --
and whether the second such field is the value of the variable ID
. If so, the next line of input is read and printed.
To use regular expressions instead,
$0 ~ "^-- " ID { getline; print }
This would match every line that starts with a double dash and a single space, followed by the value of ID
.
add a comment |
If a variable assignment is given as a file operand on the command line of an awk
script, that assignment will be carried out when awk
gets to that point in using the file operands.
Example:
awk -f script.awk a=1 file1 file2 a=2 file3 a=3 file4
Here, the awk
variable a
will be set to 1
before reading file1
. The variable will retain that value (unless it's changed by the awk
program) until the file file2
has been read. It is then set to 2
etc.
This means that your command line properly sets the variable ID
to 4
.
The difference between -v ID=4
and this is that ID
will not have been set in any BEGIN
block. Using -v ID=4
sets ID
to 4
and it will be available in BEGIN
blocks.
Another issue with your code is that you use ID
as a literal string in
if ($1 ~/--/ && $2 ~/^ID/){
And also that you seem to expect your while
loop to loop over the lines of the file. That line-by-line reading loop is already built into the way awk
operates and it very seldom needs to be explicit.
Instead,
$1 == "--" && $2 == ID { getline; print }
This will use string comparisons (not regular expression matches) to check whether the first whitespace-delimited field on the current line is the string --
and whether the second such field is the value of the variable ID
. If so, the next line of input is read and printed.
To use regular expressions instead,
$0 ~ "^-- " ID { getline; print }
This would match every line that starts with a double dash and a single space, followed by the value of ID
.
If a variable assignment is given as a file operand on the command line of an awk
script, that assignment will be carried out when awk
gets to that point in using the file operands.
Example:
awk -f script.awk a=1 file1 file2 a=2 file3 a=3 file4
Here, the awk
variable a
will be set to 1
before reading file1
. The variable will retain that value (unless it's changed by the awk
program) until the file file2
has been read. It is then set to 2
etc.
This means that your command line properly sets the variable ID
to 4
.
The difference between -v ID=4
and this is that ID
will not have been set in any BEGIN
block. Using -v ID=4
sets ID
to 4
and it will be available in BEGIN
blocks.
Another issue with your code is that you use ID
as a literal string in
if ($1 ~/--/ && $2 ~/^ID/){
And also that you seem to expect your while
loop to loop over the lines of the file. That line-by-line reading loop is already built into the way awk
operates and it very seldom needs to be explicit.
Instead,
$1 == "--" && $2 == ID { getline; print }
This will use string comparisons (not regular expression matches) to check whether the first whitespace-delimited field on the current line is the string --
and whether the second such field is the value of the variable ID
. If so, the next line of input is read and printed.
To use regular expressions instead,
$0 ~ "^-- " ID { getline; print }
This would match every line that starts with a double dash and a single space, followed by the value of ID
.
edited 13 mins ago
answered 19 mins ago


KusalanandaKusalananda
132k17252416
132k17252416
add a comment |
add a comment |
Connor Hale is a new contributor. Be nice, and check out our Code of Conduct.
Connor Hale is a new contributor. Be nice, and check out our Code of Conduct.
Connor Hale is a new contributor. Be nice, and check out our Code of Conduct.
Connor Hale is a new contributor. Be nice, and check out our Code of Conduct.
Thanks for contributing an answer to Unix & Linux Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2funix.stackexchange.com%2fquestions%2f503047%2fwriting-an-awk-script-that-reads-variable-from-command-line%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
PhYy K Vqfhs3qHaFwIjSTpOtWKT7pkrL LxTSpJFomcsI,VfC